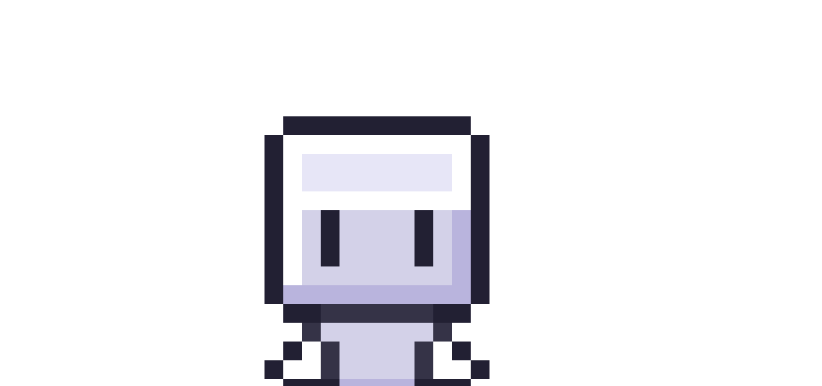
Bitfarmer Development.
I made a platformer game for the web using P5.js, Jquery, and Vanilla Javascript. Hopefully this will provide a source of entertainment for NYU students who need a distraction from their busy schedule, as well as a learning platform, if they decide to read the source code on Github.
I have always loved playing platformers from Super Mario Bros and Crash Bandicoot while growing up, to Cuphead and Hollow Knight recently. While, this project was nowhere near the caliber of those games, it was still inspiring to be able to bring something similar to life.
How to Play
Difficulty
- Easy- Low gravity for longer jumps Recommended for a casual game experience.
- Normal- Normal gravity Recommended for a challenge.
- Hard- Faster platform flickering and platform movement Recommended for experienced gamers.
Controls
- A or ← to move left.
- D or → to move right.
- W or ↑ or {SPACE} to jump.
Gameplay
Once you got the movement down, simply jump from platform to platform and collect every bitcoin on the screen. When every coin on the screen is collected, simply move the character all the way to the right, until you exit the screen, to move on to the next stage. Now just try to get through all 7 stages and collect all the coins!
Programming
Resources
- P5.js- for manipulating and drawing everything on to the HTML5 canvas.
- jQuery- for selecting elements from the DOM in order to perform the resizing functionality.
- FreeSound- For getting non-copyrighted music files.
Files
The html for the project can be found in the file index.html. This contains a div with id container which holds the canvas, a div with id p5_loading for the loading screen, as well as the elements which contain the instructions and cheatcode input. Furthermore, the index file contains some Javascript and CSS that performs most of the functionality for resizing the canvas. Next, there is the file styles.css, which holds the styling rules for our web app, including: setting the canvas width and height and the setting the loading bar style. The assets folder contains all the media used in the project, such as images, sound files, and custom fonts. Finally, there is the javascript folder called js, which contains all the logic for the web app. It holds jquery.documentsize.js which handles the rest of the functionality for resizing. Lastly, it holds the most important file: game.js, which contains the game logic.Functionality
First our game.js runs through the function preload() which loads all the media resources we need. Next it calls draw() which is called 60 times per second, causing the game elements to look like they are moving, but in actuality they are just changing each frame. Draw checks 2 different states: if the game started (if so the program draws the character, platforms, and bitcoins), or if the game has ended (if so the program draws the game won screen), finally, if neither condition is met then our program draws the main menu.
function draw(){
if(game.started){ //IN GAME
game.drawGameLoop();
}
else if(game.ended){ //END GAME
game.drawGameEndMenu();
}
else{//MAIN MENU
game.drawMenu();
}
}
The class Game, seen in the code above, holds all the drawing functions for the game (i.e game.drawMenu()) and holds the game state. I used ES6 classes for most of the game objects, such as the character, the stages, the platforms, the coins, and the scoreboard. This not only made replicating game objects, such as platforms, as easy as creating new instances, but also, helped keep the code clean.
Always code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live.
Martin Golding